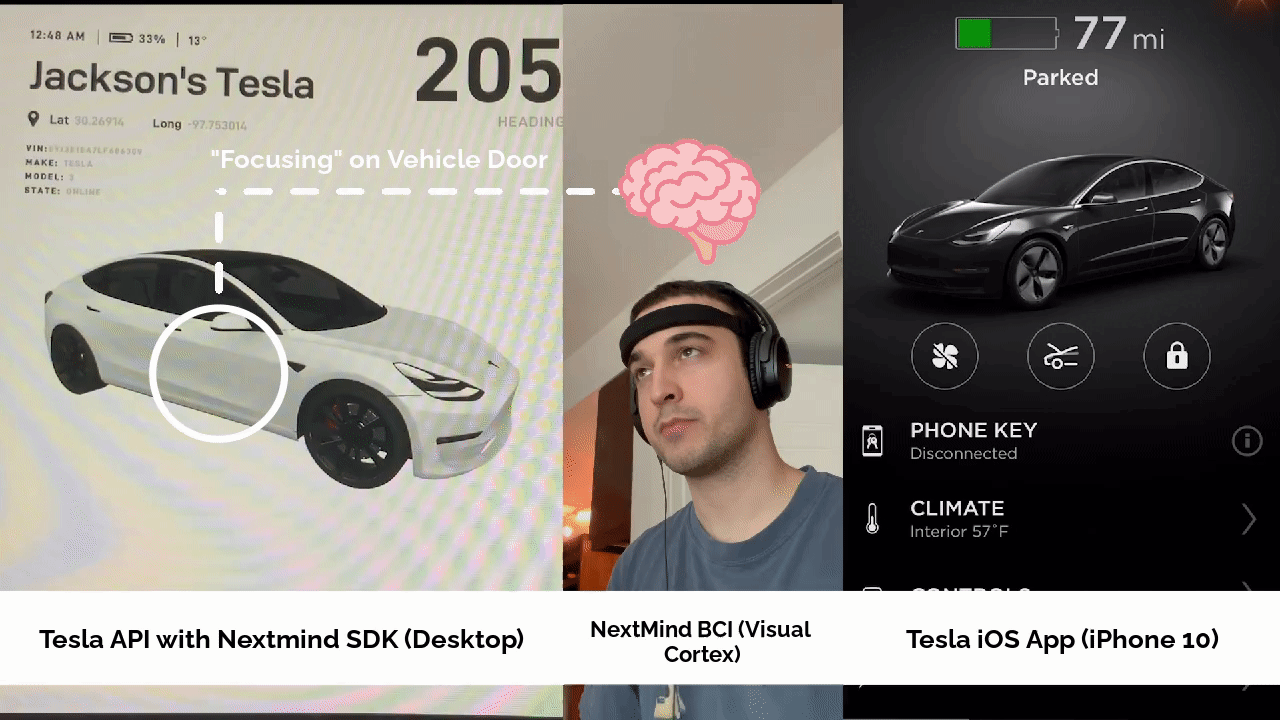
BCI-Controlled Vehicle: Tesla API Unity/C# Wrapper and NextMind BCI
Design and built a Unity/C# wrapper for Tesla's API that enables brain-controlled vehicle interactions through NextMind BCI technology, allowing users to unlock their Tesla vehicles using only their thoughts
The Tesla API Unity/C# Wrapper gives you the ability to easily use the Tesla API from your Unity applications. You can make state or command requests to your vehicle and add more endpoints for future supported features of each Tesla model. This project also contains immersive examples for creating XR applications to control your Tesla using new digital mediums. Using the NextMind BCI (Brain-Computer Interface) headset in combination with the API wrapper, I've created a neural interface that allows users to unlock their Tesla vehicles through direct brain signals. This integration demonstrates the potential of combining neural technology with vehicle automation, creating a seamless and hands-free way to interact with your Tesla.
Github: https://github.com/atx-barnes/unity-tesla-kit
Features Built
- Tesla API Wrapper for Unity (Auth2.0, List Vehicles, State / Command Requests, etc.)
- Ability to Add Vehicle Endpoint Methods for Future Vehicle Software Updates.
- Task-based Asynchronous Pattern
- Scripting API Reference Documentation
- Supported Raw Result Data (JSON)
- Supported Result Objects
- Real-time Tesla Client Console UI Examples
- Universal Render Pipeline Supported
- Control Your Tesla with Your Mind! *NextMind Headset Required
- AR Foundation Example Scene for Immersive Vehicle Control
- Cross Platform Support (MacOS, Windows, iOS, Android)
Example Usage
using UnityEngine;
using Tesla.API.Core;
public class Example : MonoBehaviour {
private async void Start() {
TeslaClient Client = this.gameObject.AddComponent<TeslaClient>();
await Client.Authenticate("your@email.com", "••••••••••••");
Client.User.GetVehicle<Model3>(async (M3) => {
var state = await M3.GetDriveStateAsync();
Debug.Log($"Vehicle Heading: {state.Response.heading}");
var command = await M3.SetChargeLimitAsync(50);
Debug.Log($"Charge Limit Set Result: {command.Response.result}");
});
}
}
Adding Vehicle Endpoints
using Tesla.API.Core;
using System.Threading.Tasks;
public static class VehicleExtensions
{
public static async Task<Command> OpenChargePort(this Vehicle vehicle) {
return await TeslaClient.Instance.MakeRequest<Command>
($"/api/1/vehicles/{vehicle.id}/command/charge_port_door_open");
}
}